How To Implement A Data Structure In Java For Bus Reservation
Domicile » C++ programs
C++ programme of Airline Seat Reservation Problem
In this article, we are going to solve a problem based on airline sit down reservation with C++ implantation.
Submitted by Radib Kar, on November 30, 2018
Problem statement: Write a program to assign passengers seats in an aeroplane. Assume a small airplane with seat numbering equally follows:
1 A B C D two A B C D three A B C D iv A B C D 5 A B C D 6 A B C D 7 A B C D
The program should display the seat pattern, with an 'Ten' marker the seats already assigned. After displaying the seats bachelor, the program prompts the seat desired, the user types in a seat, and and so the display of bachelor seats is updated. This continues until all seats are filled or until the user signals that the program should cease. If the user types in a seat that is already assigned, the program should say that the seat is occupied and ask for some other choice.
Input Instance:
For example, after seats 1A, 2B, and 4C are taken, the display should look like:
1 X B C D 2 A 10 C D three A B C D 4 A B 10 D v A B C D half dozen A B C D vii A B C D
Solution
The whole problem can be implemented with assistance of 4 major functions:
- getData()
- brandish()
- check()
- update()
The entire problem is discussed dividing into parts focusing on functional modularity.
1. Initialize a ii-D array to represent the seat matrix
A 2-D character array is used to represent the seat matrix where the first column have the row number of each seat & the rest of the columns have four seat A,B,C,D respectively. Thus it's a 7X5 ii-D assortment to represent the plane seat matrix which looks like post-obit:
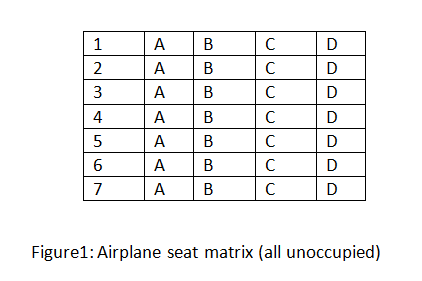
2. Take user input for seat no
User is requested to input the desired seat no by giving respective no of seat. Like a valid seat no is 1A, 3C, 7D and and so on, whereas, an invalid seat asking tin can be 6F, 0B so on.
The input is taken by our function getData() which takes user input & returns the string.
3. Check the seat no request (check() )
Our major role for this problem is to check the validity of the seat request & update seat matrix condition every bit per request.
- Firstly it checks whether the user input is in the range 1A to 7D or non. If user input is out of range a prompt out "invalid request" & go along for further asking.
- Check whether user input is 'Northward' or not. If information technology'southward 'North' then user wants to end the plan. Finish.
- if seat request is valid but already occupied
And so prompt a message stating "It's already occupied"
This checking tin be done by founding the 2-D array row & column index for the corresponding seat.
Let, row_index=r&column_index=c
If(seat_matrix[row_index][column_index]=='Ten')
Seat is occupied. - ELSE seat asking is a valid one and not occupied still
Update()
4. Update the valid entry
If the request is to update the valid seat we simple alter its value to '10'. It tin can be washed past finding row & column index and updating the value of seat_matrix at that location to '10'.
5. Special function to check whether all seats are occupied
The program too demand to exist terminated when all seats are occupied. Thus every time we keep a checking whether all the entry of seat_matrix is 'Ten' or non.
C++ implementation for Airline Seat Reservation Problem
# include < bits/stdc++.h > using namespace std ; // to bank check whether all sits are occupied or not int allOccupied( char arr[ 7 ] [ 5 ] ) { int count = 0 ; for ( int i= 0 ;i< 7 ;i+ + ) { for ( int j= 1 ;j< 5 ;j+ + ) if (arr[i] [j] = = '10' ) count + + ; } if ( count = = 28 ) return i ; render 0 ; } //to brandish the sits void brandish( char arr[ seven ] [ 5 ] ) { for ( int i= 0 ;i< 7 ;i+ + ) { for ( int j= 0 ;j< 5 ;j+ + ) { cout < <arr[i] [j] < < " " ; } cout < < endl ; } render ; } //take user information string getData( ) { string p; cout < < " enter valid seat no to bank check(like 1B) or North to cease: " ; cin > >p; return p; } //update sit status void update( char arr[ seven ] [ 5 ] , int row, int col) { cout < < " congrats, your seat is valid. Reserved for you \n " ; cout < < " updated seat status.......... \n " ; arr[row] [col] = '10' ; } //checking whether user request for //his sit down no can exist candy or non int check( char arr[ 7 ] [ 5 ] , string s) { //if user input is not in the range 1A to 7D if (southward[ 0 ] > 'vii' | | southward[ 0 ] < '1' | | south[ 1 ] > 'D' | | s[ i ] < 'A' ) { cout < < " invalid seat no \n " ; //invalid sit no return 0 ; } int row= - ane ,col= - 1 ; //find the row no of the user sit for ( int i= 0 ;i< seven ;i+ + ) { if (arr[i] [ 0 ] = =s[ 0 ] ) row=i; } //discover the column no of user sit down for ( int j= 0 ;j< 5 ;j+ + ) { if (arr[row] [j] = =south[ 1 ] ) col=j; } //cheque whether sit is already occupied or non. if (col= = - 1 ) { cout < < " Seat is already occupied \n " ; return 0 ; } else { //if it's a valid sit down & non occupied, //process the requested & update the sit equally occupied update(arr,row,col) ; } return i ; } void airline( char arr[ 7 ] [ 5 ] ) { // user tin can finish procedure by pressing 'Northward' cout < < " enter N if you are washed! \n " ; string south; // continue if not interrepted by user or //there is valid sit in unoccupied land while ( true ) { s=getData( ) ; //become user input //if user input is to terminate the process if (south[ 0 ] = = 'N' ) break ; // intermission //process the request & check according to if (check(arr,south) ) brandish(arr) ; if (allOccupied(arr) ) { //if all sits are occupied cout < < " sorry, no more than seats left!!!!!!!!!!1... " < < endl ; break ; //break } } cout < < " Thanks, that'due south all " < < endl ; //stop of program } int main ( ) { //ii-D array for storing sit down number char arr[ 7 ] [ 5 ] ; for ( int i= 0 ;i< 7 ;i+ + ) { //forst cavalcade is row number arr[i] [ 0 ] =i+ 1 + '0' ; for ( int j= 1 ;j< five ;j+ + ) { //to correspond sit number A,B,C,D respectively arr[i] [j] = 'A' +j- 1 ; } } cout < < " initial seat arrangements........ \n " ; brandish(arr) ; airline(arr) ; //airline function return 0 ; }
Output
initial seat arrangements........ 1 A B C D 2 A B C D 3 A B C D 4 A B C D 5 A B C D vi A B C D 7 A B C D enter N if you are done! enter valid seat no to check(like 1B) or N to end: 2B congrats, your seat is valid. Reserved for you updated seat condition.......... 1 A B C D 2 A X C D 3 A B C D 4 A B C D 5 A B C D six A B C D vii A B C D enter valid seat no to check(like 1B) or Northward to end: 3C congrats, your seat is valid. Reserved for yous updated seat status.......... 1 A B C D 2 A 10 C D 3 A B 10 D 4 A B C D 5 A B C D 6 A B C D 7 A B C D enter valid seat no to check(similar 1B) or N to end: 2B Seat is already occupied enter valid seat no to check(like 1B) or N to end: 7E invalid seat no enter valid seat no to check(similar 1B) or N to end: 7C congrats, your seat is valid. Reserved for yous updated seat status.......... ane A B C D 2 A Ten C D three A B X D iv A B C D 5 A B C D 6 A B C D 7 A B X D enter valid seat no to cheque(like 1B) or N to stop: N Cheers, that'south all
Ad
Advertizing
How To Implement A Data Structure In Java For Bus Reservation,
Source: https://www.includehelp.com/cpp-programs/airline-seat-reservation-problem.aspx
Posted by: daughtreymuchey.blogspot.com
0 Response to "How To Implement A Data Structure In Java For Bus Reservation"
Post a Comment